JAVA/연습문제
쉽게 배우는 자바 프로그래밍 2판 10장 도전과제
세언이
2022. 11. 1. 18:57
반응형
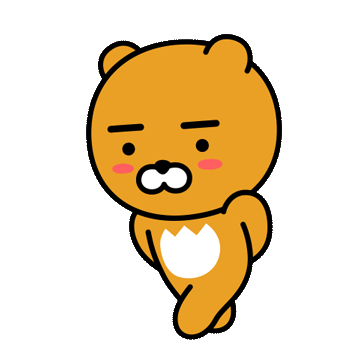
# 개발 공부하는 초보자가 작성한 답안이니 정답이 아닐 수 있습니다!!!
# 주어진 다수의 도형에서 특정 도형을 찾아내는 프로그램을 다음과 같이 세 가지 방식, 즉 다수의 메서드, 모든 경우를 매개변수로 추가한 메서드, 람다식을 이용한 메서드로 작성한 후 각 방식을 비교해보자.
1. 다음 과정에 따라 Shape 클래스와 테스트 프로그램을 완성하자.
1-1. Shape 클래스는 다음과 같은 필드, 모든 필드를 초기화하는 생성자, 모든 필드에 대한 getter 메서드를 가진다. 그리고 '도형타입(색깔, 면적)'을 반환하는 toString() 메서드도 있다.
📢주어진 값📢
private String type; // 도형의 종류
private String color; // 도형의 색깔
private Double area; // 도형의 면적
🧤정답🧤
public class Shape {
private String type;
private String color;
private Double area;
public Shape(String type, String color, Double area) {
this.type = type;
this.color = color;
this.area = area;
}
public String getColor() {
return color;
}
public String getType() {
return color;
}
public Double getArea() {
return area;
}
public String toString() {
return "도형 타입 : " + type + "(" + color + ", " + area + ")";
}
}
1-2. 테스트 프로그램에서 사용할 정적 ArrayList<Shape> 타입의 shapes를 Shape 클래스에 다음과 같이 정의한다.
📢주어진 값📢
삼각형, 빨간색, 10.5
사각형, 파란색, 11.2
원, 파란색, 16.5
원, 빨간색, 5.3
원, 노란색, 8.1
사각형, 파란색, 20.7
삼각형, 파란색, 3.4
사각형, 빨간색, 12.6
🧤정답🧤
public static final ArrayList<Shape> shapes = new ArrayList<>(Arrays.asList(new Shape("삼각형", "빨간색", 10.5),
new Shape("사각형", "파란색", 11.2), new Shape("원", "파란색", 16.5), new Shape("원", "노란색", 8.1),
new Shape("사각형", "파란색", 20.7), new Shape("삼각형", "파란색", 3.4), new Shape("사각형", "빨간색", 12.6)));
1-3. 특정 도형을 찾기 위하여 다음과 같은 두 메서드를 구현한 ShapeTest 클래스를 작성한다.
📢주어진 조건📢
static List<Shape> findShapesByType(List<Shape> shapes, String type)
static List<Shape> findShapesByColorNArea(List<Shape> shapes, String color, double area)
🧤정답🧤
static List<Shape> findShapesByColorNArea(List<Shape> shapes, String color, double area) {
List<Shape> result2 = new ArrayList<>();
for (Shape colorArea : shapes) {
if (colorArea.getColor().equals(color) && colorArea.getArea().equals(area)) {
result2.add(colorArea);
}
}
return result2;
}
static List<Shape> findShapesByType(List<Shape> shapes, String type) {
List<Shape> result = new ArrayList<>();
for (Shape shapeType1 : shapes) {
if (shapeType1.getType().equals(type))
result.add(shapeType1);
}
return result;
}
1-4. 다음과 같은 2가지 실행 결과(사각형, 면적이 12.0이하인 빨간색 도형)가 나타나도록 main() 메서드를 작성하라.
📢주어진 결과📢
사각형 : [사각형(파란색, 11.2), 사각형(파란색, 20.7), 사각형(빨간색, 12.6)]
빨간 도형(면적<=12.0) : [삼각형(빨간색, 10.5), 원(빨간색, 5.3)]
🧤정답🧤
public class Shape {
private String type;
private String color;
private Double area;
public Shape(String type, String color, Double area) {
this.type = type;
this.color = color;
this.area = area;
}
public String getColor() {
return color;
}
public String getType() {
return type;
}
public Double getArea() {
return area;
}
public String toString() {
return type + "(" + color + ", " + area + ")";
}
// Arrays.asList를 통해 List형태로 변환
public static final ArrayList<Shape> shapes = new ArrayList<>(
Arrays.asList(new Shape("삼각형", "빨간색", 10.5), new Shape("사각형", "파란색", 11.2), new Shape("원", "파란색", 16.5),
new Shape("원", "빨간색", 5.3), new Shape("원", "노란색", 8.1), new Shape("사각형", "파란색", 20.7),
new Shape("삼각형", "파란색", 3.4), new Shape("사각형", "빨간색", 12.6)));
// 위와 같은 코드
// public static final List<Shape> shapes = Arrays.asList(new Shape("삼각형", "빨간색", 10.5), new Shape("사각형", "파란색", 11.2),
// new Shape("원", "파란색", 16.5), new Shape("원", "노란색", 8.1), new Shape("사각형", "파란색", 20.7),
// new Shape("삼각형", "파란색", 3.4), new Shape("사각형", "빨간색", 12.6));
}
public class ShapeTest {
public static void main(String[] args) {
List<Shape> circlefindGabType = findShapesByType(Shape.shapes, "사각형");
System.out.println("사각형 : " + circlefindGabType);
List<Shape> colorAreafindGabType = findShapesByColorNArea(Shape.shapes, "빨간색", 12.0);
System.out.println("빨간 도형(면적<=12.0) : " + colorAreafindGabType);
}
public static List<Shape> findShapesByColorNArea(List<Shape> shapes, String color, double area) {
List<Shape> result2 = new ArrayList<>();
for (Shape colorArea : shapes) {
if (colorArea.getColor().equals(color) && colorArea.getArea() < area) {
result2.add(colorArea);
}
}
return result2;
}
public static List<Shape> findShapesByType(List<Shape> shapes, String type) {
List<Shape> result = new ArrayList<>();
for (Shape shapeType1 : shapes) {
if (shapeType1.getType().equals(type))
result.add(shapeType1);
}
return result;
}
}
2. 1번과 동일한 실행 결과가 나타나도록 모든 경우에 사용할 수 있는 통합된 메서드를 작성 해보자.
2-1. 1-3에서 작성한 findShapesByType()과 findShapesByColorNArea()의 모든 매개 변수를 가진 다음 메서드를 작성한다.
📢주어진 조건📢
static List<Shape> findShapes(List<Shape> shapes, String type, String color, Double area)
🧤정답🧤
public static List<Shape> findShapes(List<Shape> shapes, String type, String color, Double area) {
List<Shape> result3 = new ArrayList<>();
for (Shape typeColorArea : shapes) {
if (typeColorArea.getType().equals(type)) {
result3.add(typeColorArea);
} else if (typeColorArea.getColor().equals(color) && typeColorArea.getArea() < area) {
result3.add(typeColorArea);
}
}
return result3;
}
2-2. 1-4의 실행 결과와 동일하도록 main() 메서드를 작성한다.
🧤정답🧤
public class ShapeTest {
public static void main(String[] args) {
List<Shape> circlefindGabType = findShapes(Shape.shapes, "사각형", "", 1.0);
System.out.println("사각형 : " + circlefindGabType);
List<Shape> colorAreafindGabType = findShapes(Shape.shapes, "", "빨간색", 12.0);
System.out.println("빨간 도형(면적<=12.0) : " + colorAreafindGabType);
}
public static List<Shape> findShapes(List<Shape> shapes, String type, String color, Double area) {
List<Shape> result3 = new ArrayList<>();
for (Shape typeColorArea : shapes) {
if (typeColorArea.getType().equals(type)) {
result3.add(typeColorArea);
} else if (typeColorArea.getColor().equals(color) && typeColorArea.getArea() < area) {
result3.add(typeColorArea);
}
}
return result3;
}
}
3. 1번과 2번은 다수의 유사한 메서드 사용, 필요 없는 매개 변수 사용 등으로 가독성이 떨어지며 코드도 지저분하다. 또한, 다른 특징의 도형을 찾는다면 새로운 메서드를 추가하거나 혹은 더 많은 매개변수를 포함하는 메서드로 수정해야 한다. 이와 같은 문제점을 제거하기 위하여 함수형 인터페이스와 람다식으로 작성해 보자.
3-1. 다음과 같은 함수형 인터페이스 타입을 사용하는 메서드를 구현한다.
📢주어진 값📢
static List<Shape> findShapes(List<Shape> shapes, Predicate<Shape> p)
🧤정답🧤
public static List<Shape> findShapes(List<Shape> shapes, Predicate<Shape> p) {
List<Shape> result2 = new ArrayList<>();
for (Shape typeColorArea : shapes) {
if (p.test(typeColorArea)) {
result2.add(typeColorArea);
}
}
return result2;
}
3-2. 1번의 실행 결과와 동일하도록 main() 메서드를 작성한다.
🧤정답🧤
public interface Predicate<Shape> {
boolean test(Shape shape);
}
public class ShapeTest {
public static void main(String[] args) {
List<Shape> circlefindGabType = findShapes(Shape.shapes, c -> c.getType().equals("사각형"));
System.out.println("사각형 : " + circlefindGabType);
List<Shape> colorAreafindGabType = findShapes(Shape.shapes,
c -> c.getColor().equals("빨간색") && c.getArea() <= 12.0);
System.out.println("빨간 도형(면적<=12.0) : " + colorAreafindGabType);
}
public static List<Shape> findShapes(List<Shape> shapes, Predicate<Shape> p) {
List<Shape> result2 = new ArrayList<>();
for (Shape typeColorArea : shapes) {
if (p.test(typeColorArea)) {
result2.add(typeColorArea);
}
}
return result2;
}
}
반응형