JAVA/연습문제
쉽게 배우는 자바 프로그래밍 2판 9장 프로그래밍 문제 Part.1 (1번 ~ 5번)
세언이
2022. 10. 28. 17:05
반응형
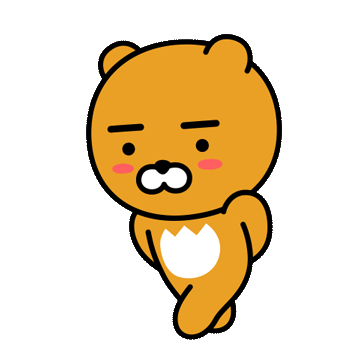
# 개발 공부하는 초보자가 작성한 답안이니 정답이 아닐 수 있습니다!!!
1. 다음과 같은 테스트 프로그램과 실행 결과가 있다. 실행 결과가 나타나도록 MyDate 클래스를 작성하고, 테스트 프로그램의 오류도 수정하라.
📢주어진 조건📢
public class NullPointerExceptionTest {
public static void main(String[] args) {
MyDate d = null;
System.out.printf("%d년 %d월 %d일\n", d.year, d.month, d.day);
}
}
📢주어진 결과값📢
2035년 12월 25일
🧤정답🧤
public class NullPointerExceptionTest {
public static void main(String[] args) {
MyDate d = new MyDate();
System.out.printf("%d년 %d월 %d일\n", d.year, d.month, d.day);
}
}
public class MyDate {
int year = 2035;
int month = 12;
int day = 25;
}
2. 다음 프로그램을 실행하면 castind() 메서드가 예외를 발생시킨다. 예외의 원인을 조사하라. 그리고 casting() 메서드에서 발생한 예외를 메인 메서드가 처리하도록 프로그램을 수정하라.
📢주어진 조건📢
class Shape {}
class Rectangle extends Shape{}
class Circle extends Shape{}
public class CastExceptionTest {
public static void main(String[] args) {
Rectangle r = new Rectangle();
casting(r);
}
static void casting(Shape s) throws ClassCastException{
Circle c = (Circle) s;
}
}
🧤정답🧤
class Shape {}
class Rectangle extends Shape{}
class Circle extends Shape{}
public class CastExceptionTest {
public static void main(String[] args) {
Rectangle r = new Rectangle();
try {
casting(r);
}
catch (ClassCastException d) {
d.printStackTrace();
System.out.println("잘못된 형변환입니다.");
}
}
static void casting(Shape s) throws ClassCastException{
Circle c = (Circle) s;
}
}
3. 다음 프로그램과 실행 결과를 보고 showTokens() 메서드를 완성하라.
📢주어진 조건📢
public class TokenPrintTest {
public static void main(String[] args) {
String s = "of the people, by the people, for the people";
try {
showTokens(s, ", ");
} catch (NoSuchElementException e) {
System.out.println("끝");
}
}
// while(true) {}을 사용하는 showTokens() 메서드 추가
}
📢주어진 결과값📢
of
the
people
by
the
people
for
the
people
끝
🧤정답🧤
import java.util.NoSuchElementException;
import java.util.StringTokenizer;
public class TokenPrintTest {
public static void main(String[] args) {
String s = "of the people, by the people, for the people";
try {
showTokens(s, ", ");
} catch (NoSuchElementException e) {
System.out.println("끝");
}
}
// 비검사형이여서 showTokens에 throws NoSuchElementException을 안 붙여줘도 된다.
public static void showTokens(String s, String s2) throws NoSuchElementException {
StringTokenizer st = new StringTokenizer(s, s2);
while (true) {
System.out.println(st.nextToken());
}
}
}
4. 다음 프로그램과 실행 결과를 참고해 Pair 클래스를 작성하라. Pair 클래스는 2개의 필드와 2개의 메서드를 가진다. 2개의 필드는 숫자를 나타내는 어떤 타입도 될 수 있고, 2개의 메서드는 first()와 second()로 각각 첫 번째 필드 값, 두 번째 필드 값을 반환한다.
📢주어진 조건📢
public class PairTest {
public static void main(String[] args) {
Pair<Integer> p1 = new Pair<>(10,20);
Pair<Double> p2 = new Pair<>(10.0,20.0);
System.out.println(p1.first());
System.out.println(p2.second());
}
}\
📢주어진 결과값📢
10
20.0
🧤정답🧤
public class PairTest {
public static void main(String[] args) {
Pair<Integer> p1 = new Pair<>(10,20);
Pair<Double> p2 = new Pair<>(10.0,20.0);
System.out.println(p1.first());
System.out.println(p2.second());
}
}
public class Pair <Number>{
Number x, y;
public Pair (Number x, Number y) {
this.x = x;
this.y = y;
}
public Number first() {
return x;
}
public Number second() {
return y;
}
}
5. 다음은 모든 동물의 소리를 출력하는 제네릭 메서드를 테스트하는 프로그램이다. 2개의 동물(Dog와 Cuckoo) 클래스를 정의하고, 제네릭 메서드 printSound()를 완성하라.
📢주어진 조건 1📢
interface Animal {
void sound();
}
// Dog 클래스와 Cuckoo 클래스
public class AnimalSoundTest {
// printSound() 메서드
public static void main(String[] args) {
List<Animal> lists = new ArrayList<>();
lists.add(new Dog());
lists.add(new Cuckoo());
printSound(lists);
List<Dog> dogs = new ArrayList<>();
dogs.add(new Dog());
printSound(dogs);
}
}
📢주어진 결과값📢
멍멍~~
뻐꾹뻐꾹~~
멍멍~~
🧤정답🧤
import java.util.ArrayList;
import java.util.List;
interface Animal {
void sound();
}
class Dog implements Animal {
public void sound() {
System.out.println("멍멍~");
}
}
class Cuckoo implements Animal {
public void sound() {
System.out.println("뻐꾹뻐꾹~~");
}
}
public class AnimalSoundTest {
static void printSound(List<? extends Animal> lists) {
for (Animal randomAnimal : lists) {
randomAnimal.sound();
}
}
public static void main(String[] args) {
List<Animal> lists = new ArrayList<>();
lists.add(new Dog());
lists.add(new Cuckoo());
printSound(lists);
List<Dog> dogs = new ArrayList<>();
dogs.add(new Dog());
printSound(dogs);
}
}
반응형